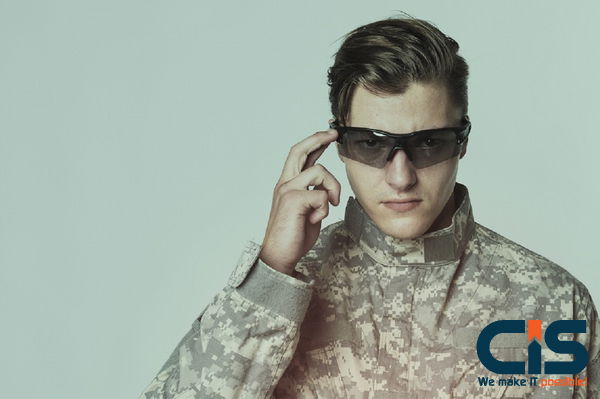
According to Statista, the global market for application development software is anticipated to generate an astounding US$195.77 billion in revenue by 2025. In this blog, we'll explore the .NET framework's relevance in today's application development.
The .NET applications are categorized into various types such as desktop applications, which focus on Windows-based software, web applications for creating dynamic websites and APIs, mobile applications through Xamarin and MAUI for cross-platform development, and cloud applications utilizing Azure for scalable and secure solutions.
The ecosystem comprises .NET Core, which is open-source and cross-platform, the .NET Framework suited for Windows-specific projects, and Xamarin for cross-platform mobile development.
Read Also: Why .NET Frameworks for Dynamic Apps? Maximize Your Impact with Cost-Effective Development!
Setting Up Your Development Environment
Building a .NET application begins with preparing your development environment. Here's how to do it effectively:
Prerequisites for .NET Development
Before diving into development, ensure your system meets the requirements:
- Operating System: Windows, macOS, or Linux
- RAM: Minimum of 4GB, though 8GB is recommended
- Processor: 1.8 GHz or faster
- Disk Space: At least 10GB for tools and libraries
Choosing Your Integrated Development Environment (IDE):
Selecting the right IDE is crucial for a smooth development experience. Here are some options:
Visual Studio:
- A comprehensive, feature-rich environment is ideal for full-fledged .NET development.
- Offers built-in tools for debugging, testing, and deployment.
Visual Studio Code:
- A lightweight, cross-platform editor perfect for simpler tasks.
- Strong support for .NET through extensions like C#.
JetBrains Rider:
- An advanced, cross-platform IDE with smart coding assistance.
- Offers powerful refactoring and navigation features.
Installing the Necessary SDKs:
Download and install the appropriate SDKs based on your development needs:
.NET Core:
- Ideal for cross-platform applications.
- Suitable for developing modern, cloud-based applications.
.NET Framework:
- Best for Windows-based applications.
- Provides extensive class libraries and a robust runtime environment.
With these steps, your development environment will be ready, making the next phases of building .NET application much smoother. Whether you're targeting web applications or desktop software, setting up correctly is key to success. This foundation ensures you have the tools necessary to bring your application ideas to life.
Understanding the .NET Architecture
The .NET architecture is essential for anyone looking to develop robust applications. Here's a breakdown:
- Common Language Runtime (CLR): CLR plays a central role in executing .NET applications. It handles memory management, ensuring that resources are efficiently allocated and freed. This minimizes the chances of memory leaks, making your applications run smoothly.
- Intermediate Language (IL): When you write code in any .NET language, it gets compiled into an Intermediate Language. This IL is then converted into machine code that your computer can execute. This process makes .NET applications fast and responsive.
- .NET Standard: This is a set of base class libraries that allows your code to be compatible across different platforms. By adhering to .NET standards, you ensure your applications can run on Windows, macOS, Linux, and more without any hiccups.
- Assemblies and Global Assembly Cache (GAC): Assemblies are the building blocks of .NET applications. They contain compiled code and resources. The Global Assembly Cache stores these assemblies for shared use across different applications, managing dependencies efficiently.
Understanding these components of .NET architecture is crucial for creating applications that are performant, portable, and scalable. This knowledge ensures you can build applications that are versatile and user-friendly.
Creating Your First .NET Application
Creating your first .NET application can be a thrilling experience. Let's dive into a step-by-step guide that will walk you through building a simple console application, the cornerstone for mastering .NET development.
Initialize a .NET Project:
- Using Command Line: Open the command prompt, navigate to your desired directory, and type dotnet new console -n MyFirstApp. This command launches a brand-new console program called "MyFirstApp."
- Using an IDE: If you prefer a graphical interface, you can use Visual Studio. Start by selecting "Create a new project," choose "Console App (.NET Core)," and follow the prompts.
Write and Execute a "Hello World" Program:
- Open the Program.cs file. You will see some pre-generated code. Locate the Main method and add Console.WriteLine("Hello, World!");.
- To run your application, return to the command line and type dotnet run within your project directory, or press F5 in Visual Studio.
Understand the Project Structure:
- Program.cs: This is where your main application logic resides.
- .csproj File: Contains project settings and configurations that .NET uses to build your application.
By following these steps, you'll have a working create ASP.NET application, providing a solid foundation to explore further .NET features.
Developing a Web Application with ASP.NET Core
Creating web applications with ASP.NET Core offers numerous advantages such as exceptional performance and scalability. ASP.NET Core is a powerful and flexible framework that helps developers build dynamic, responsive web applications efficiently.
To begin developing a basic ASP.NET Core MVC application, you'll need to understand the fundamental components: models, views, and controllers.
- Models: These are classes that represent the data and business logic of your application. They help in managing the data and validations.
- Views: These are the user interface parts where the HTML content is generated. Razor Pages, a new feature in ASP.NET Core, allows for creating rich, dynamic content easily.
- Controllers: These handle the request and response cycle, linking users' input with the views and models.
One of the standout features of ASP.NET Core is its built-in dependency injection system. Dependency injection helps in writing cleaner, more maintainable code by reducing tight coupling and making components more testable.
By using ASP.NET Core's powerful features and best practices, you can build fast, scalable, and secure web applications. Its flexibility allows your apps to grow with your business, ensuring a smooth user experience and efficient development, helping you stay competitive in a fast-changing market.
Building Desktop Applications with .NET
Building .NET applications involves using powerful frameworks like Windows Forms and Windows Presentation Foundation (WPF). These tools enable developers to build intuitive and interactive applications for Windows environments.
- Windows Forms: This is a GUI class library within .NET that lets you develop feature-rich desktop applications. It's perfect for those who need straightforward, form-based user interfaces with easy handling of user inputs.
- Windows Presentation Foundation (WPF): WPF provides a more modern approach, allowing developers to create visually appealing applications. It supports rich media, animations, and enhanced control over the appearance of the interface.
To start building a desktop application with .NET:
- Use Visual Studio: This integrated development environment is ideal for creating .NET applications. It offers a comprehensive set of tools that simplify the development process.
- Design the User Interface: Utilize drag-and-drop tools in Visual Studio to design your application's UI quickly.
- Handle Events and User Interactions: Write code to manage user inputs, responses, and other interactions within the application.
- Deploy the Application: Once your application is ready, deploy it using Visual Studio's built-in packaging and deployment features.
With these steps, you can effectively create desktop applications using .NET and Visual Studio.
Cross-Platform Mobile App Development with Xamarin
Xamarin is a powerful tool in the .NET ecosystem that allows developers to create mobile applications that run on multiple platforms, such as Android and iOS, using a single codebase. Here's how you can start leveraging Xamarin for your app development:
Overview of Xamarin:
Xamarin integrates seamlessly with the .NET framework, enabling developers to use C# to write native applications. This approach ensures that apps have the native performance and look of platform-specific applications.
Setting Up a Xamarin Project:
- Start by installing Xamarin in Visual Studio, which offers templates to kickstart your project.
- Configure your development environment to handle both Android and iOS platforms. This typically involves setting up the Android SDK and Xcode for iOS.
Sharing Code Across Platforms:
- Utilize .NET MAUI (Multi-platform App UI) to share business logic and user interface code between Android and iOS. This reduces development time and maintains code consistency.
Leveraging Platform-Specific Features:
- Xamarin allows access to native APIs and functionalities, such as camera controls, GPS, and other device-specific features, ensuring your app utilizes each platform's unique capabilities.
- Xamarin simplifies the development of cross-platform applications, making it a robust choice for creating versatile and responsive mobile applications.
Advanced Topics in .NET Development
Delve deeper into .NET development by exploring advanced features that can enhance your applications. Here are some key areas to focus on:
- Entity Framework Core: Simplify your data access with this powerful object-relational mapping (ORM) tool. It allows developers to work with databases using .NET objects, eliminating complex SQL code. This boosts productivity and maintains a cleaner codebase.
- Authentication and Authorization: Implementing secure login systems is crucial for any application. .NET Identity provides robust tools for managing user credentials and permissions, ensuring only authorized users access your apps. This enhances security and builds user trust.
- Using Third-Party Libraries: Extend the functionality of your applications by integrating NuGet packages. These libraries offer pre-built solutions that save development time and provide additional features without starting from scratch. NuGet integration is seamless, making it an excellent choice for .NET developers.
Mastering these advanced topics can significantly improve the efficiency and security of your .NET applications. Embrace these technologies to develop robust, high-performing software and stand out in the competitive tech landscape. Stay ahead by leveraging these advanced .NET development strategies for more efficient applications.
Testing and Deployment of .NET Applications
Testing and deploying .NET applications effectively is critical to ensure they perform flawlessly in various environments. Here's how to streamline the process:
- Unit Testing: Implement automated tests to catch bugs early. Utilize popular frameworks such as MSTest, NUnit, and xUnit to write and execute these tests efficiently. These tools help ensure that each component of your .NET application functions as intended, providing a robust testing environment.
- Continuous Integration and Deployment (CI/CD): Leverage the power of CI/CD to automate your build, test, and deployment processes. Use platforms like Azure DevOps or GitHub Actions to integrate these practices seamlessly into your development workflow. This automation helps to detect and fix issues quickly, reducing deployment times and improving code quality.
- Deployment: Deploy your .NET applications using reliable hosting and scaling solutions like IIS, Azure, or other trusted cloud providers. These platforms offer powerful features to support application scaling and performance management, ensuring an optimized end-user experience.
By focusing on these steps, you can enhance the reliability, performance, and user satisfaction of your .NET applications. Implementing automated testing and streamlined deployment practices not only boosts efficiency but also aligns with industry best practices.
Read More:Maximize Your Impact: Become a Great .NET Developer in this year with These Tips!
Resources and Further Learning
If you're looking to deepen your knowledge of .NET development, here are some valuable resources and opportunities to consider:
- Microsoft's Official .NET Documentation: This is your go-to resource for comprehensive information on every aspect of .NET. It's regularly updated and covers everything from beginner tips to advanced features.
- Online Courses: Platforms like Pluralsight, Udemy, and Coursera offer a wide range of .NET courses. These courses vary in complexity, catering to both novices and seasoned developers looking to refine their skills.
- GitHub Repositories: Explore sample .NET projects on GitHub. These repositories provide practical examples of how different features and functionalities can be implemented in real-world .NET applications.
- Join the .NET Community: Engage with other developers on platforms like Stack Overflow and GitHub. These forums are perfect for asking questions, sharing insights, and finding solutions to common challenges.
- Meetups and Conferences: Attend .NET meetups and conferences. These events provide insightful talks and valuable networking opportunities, helping you stay updated on the latest trends and innovations in .NET development.
Exploring these resources will strengthen your .NET skills and keep you connected with the wider developer community.
Conclusion
Building .NET applications involves understanding a few key steps: setting up your development environment, mastering C# or VB.NET, and utilizing the vast libraries and frameworks available.
Starting with simple projects allows you to gradually build your skills and take on more complex challenges. The .NET platform offers incredible versatility and power, making it an excellent choice for developing a wide range of applications, from desktop and web apps to mobile solutions.
As you refine your craft, you'll appreciate the robust tools and community support that .NET provides. If you're ready to embark on your development journey or need expert guidance, CISIN is here to help transform your ideas into fully functional .NET applications. Contact us today to get started.
Frequently Asked Questions (FAQs)
- What are the main differences between the .NET Core and the .NET Framework?This question addresses the fundamental distinctions between these two variants, including their platform support, intended use cases, and performance characteristics.
- What are some ways to make my.NET apps run faster?Exploring various strategies and best practices for performance optimization within .NET applications, such as efficient memory use, choosing appropriate data structures, and leveraging asynchronous programming.
- What role do microservices play in .NET application development?An explanation of how microservices architecture can be implemented in .NET, and how it can enhance the scalability and maintainability of applications.
- How do I integrate machine learning functionalities into .NET applications?Guidance on adding machine learning capabilities to .NET applications, including libraries such as ML.NET and integration techniques.
- What are some common security best practices for .NET developers?Discussing important security measures to protect .NET applications from vulnerabilities, including authentication practices, data encryption, and regular updates.
- How can I effectively manage and version control my .NET projects?Insights into using tools like Git for version control, as well as strategies for managing dependencies and project versioning within .NET environments.
Create High-Performing .NET Applications with CISIN
Partner with CISIN to create a .NET application. Our expert developers will help you build fast, scalable, and secure solutions tailored to your business needs. Get started with CISIN today!